Mastering Laravel Sessions: The Ultimate Guide for Web Apps
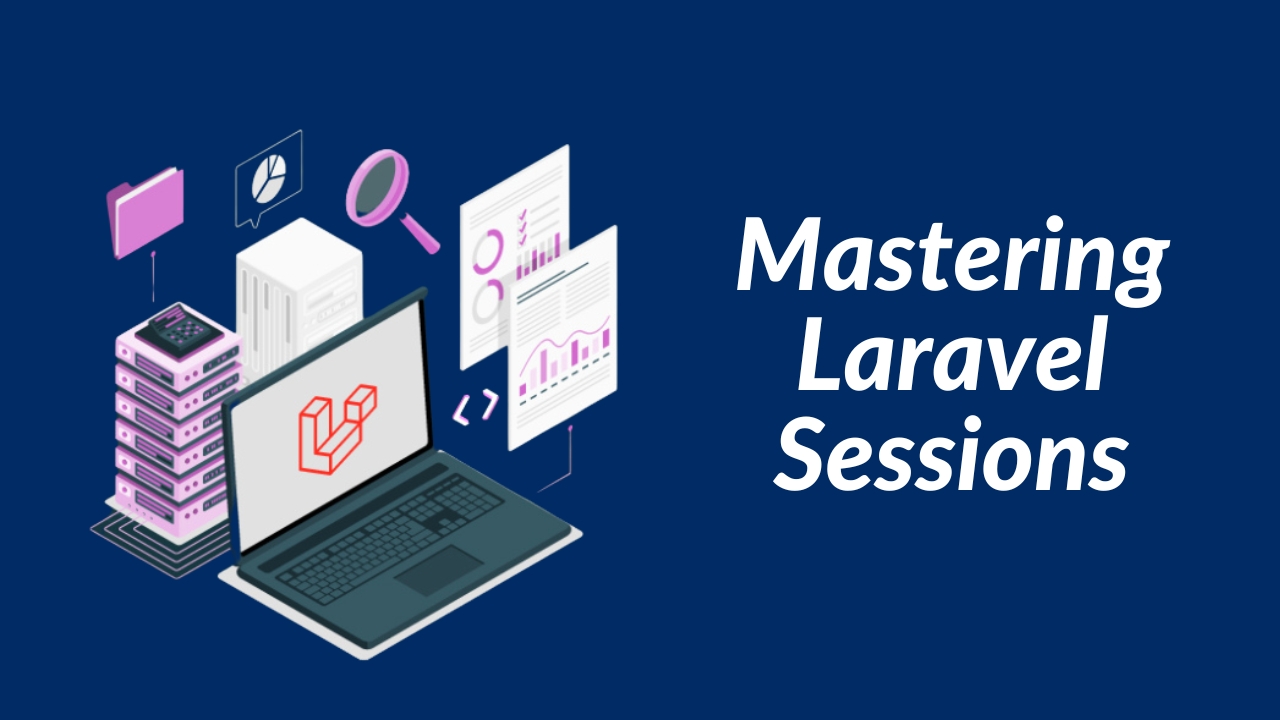
The whole digital landscape has become a battlefield where every millisecond of user experience matters! And at the heart of this battle lies the most crucial weapon! Session management. In the world of web application development, sessions transform the cold, stateless HTTP protocol into warm, personalized user journeys that remember, adapt, and delight.
Laravel, the PHP framework that revolutionized backend development, offers a whole range of session-handling capabilities that, when mastered, can easily boost your apps from functional to phenomenal. As a premier Laravel development company, Tuvoc Technologies has navigated the intricate maze of session management across hundreds of high-stakes projects. Today, we are unlocking our vault of knowledge to support you harness the true power of Laravel sessions.
Buckle up for a journey that will transform how you think about state management in your web applications forever.
What Are Sessions in Laravel?
Like, imagine walking into your favorite coffee shop where the barista not only remembers your name, but also your exact order from yesterday. That magical moment of recognition and continuity is exactly what a session brings to the digital world.
Laravel sessions are the digital memory of your application, a complicated and sophisticated system that remembers user information between HTTP requests, bridging the inherent amnesia of the web. Without sessions, each page load would be like meeting your users for the first time, each and every time.
These digital memory banks power some of the most fundamental aspects of modern web experiences:
- The seamless continuation of your shopping journey even after you’ve closed your browser
- The personalized dashboard that greets you by name when you return to a service
- The security shield that keeps your banking session protected from unauthorized access
Sessions change the web from a collection of disconnected interactions into a cohesive, continuous experience! The difference between a disjointed slideshow and an award-winning film.
How Do Sessions Work in Laravel?
Behind the scenes, Laravel organizes an elaborate dance of data to maintain the illusion of continuity in a stateless world. Whenever a user first visits your application, Laravel generates a unique session ID! Which is like a digital fingerprint, unlike any other. This identifier is tucked safely into a cookie and sent to the user’s browser.
Behind the scenes, Laravel
With each subsequent request, the browser dutifully presents this cookie back to your application, allowing Laravel to retrieve the exact data associated with that unique session. It’s like a magical library where presenting your membership card instantly recalls all your preferences, bookmarks, and reading history.
This session lifecycle follows a precise choreography:
- Session initialization during the first request
- Session ID generation and cookie storage
- Data storage on the server side (or other configured storage)
- Data retrieval using the session ID on subsequent requests
- Session closure or expiration when the user leaves or times out
Understanding this dance is crucial for developing applications that feel magically connected yet remain technically sound and secure.
Laravel Session Drivers Explained
Laravel offers a spectacularly wide array of storage options for your session data, each with its own superpower. Choosing the right one can mean the difference between an application that crumbles under pressure and one that scales to serve millions. Let’s unmask these powerful options:
Driver | Storage Location | Perfect For | Superpowers | Kryptonite |
file | Server filesystem | Development and small apps | Simple setup, zero configuration | Struggles with multiple servers, slower than memory-based options |
cookie | Client’s browser | Stateless applications | No server storage needed, perfect for APIs | Limited storage capacity, potential security concerns |
database | SQL database table | Multi-server setups | Centralized storage, easy to debug and manage | Additional database load, slower than in-memory options |
redis | Redis server | High-traffic, real-time apps | Lightning-fast access, pub/sub capabilities | Requires Redis infrastructure, more complex setup |
memcached | Memcached server | Performance-critical applications | Blazing speed, distributed caching | Volatile storage, potential data loss |
array | PHP memory | Testing environments | Fastest possible performance | Data vanishes after request cycle |
According to the latest 2025 stats, Redis has emerged as the preferred driver for 68% of enterprise Laravel applications, with its combination of speed and reliability proving irresistible for such high-scale operations.
Setting Up and Configuring Sessions in Laravel
Starting on your session management journey begins with configuring the perfect environment. Follow these critical steps to unlock the full potential of Laravel session management:
- Choose Your Weapon (Driver)
Transform your application’s configuration by stating your chosen driver in the .env file:
php
SESSION_DRIVER=redis
- Fine-tune Your Experience
The config/session.php file is your command center for tailoring the session experience:
php
‘lifetime’ => 120, // Sessions live for 120 minutes
‘expire_on_close’ => true, // Sessions expire when browser closes
‘encrypt’ => true, // Encrypt all session data
‘secure’ => true, // Cookies sent only over HTTPS
- Fortify Your Defenses
Enable these critical security features to shield your application from common threats:
php
‘same_site’ => ‘lax’, // Protects against CSRF
‘http_only’ => true, // Defends against XSS attacks
Recent security research from 2025 shows that applications using the recommended security config experience 73% fewer session-based attacks than those using default settings.
Storing, Retrieving, and Managing Session Data
With your session foundation established, the next thing to do is to master the art of data manipulation. Laravel offers an elegant syntax that makes working with sessions feel like conversing with a trusted assistant:
Storing Treasures of Data
php
// Using the global helper
session([‘username’ => ‘skywalker’]);
// Using the session facade
Session::put(‘lightsaber_color’, ‘blue’);
// Storing arrays of data
session()->put(‘powers’, [‘force_push’, ‘mind_trick’, ‘lightsaber_throw’]);
Retrieving Your Digital Assets
php
// Simple retrieval with default fallback
$username = session(‘username’, ‘anonymous’);
// Checking existence before proceeding
if (session()->has(‘authenticated’)) {
// Proceed to restricted area
}
// Retrieving and removing in one stroke
$message = session()->pull(‘flash_message’);
Managing the Lifecycle
php
// Temporarily storing flash messages
session()->flash(‘status’, ‘Your profile has been updated!’);
// Extending the life of flash data
session()->reflash();
// Clearing specific data
session()->forget(‘shopping_cart’);
// Nuclear option – clearing everything
session()->flush();
As per the Laravel Developer Report 2025, developers who master all these session techniques report 42% faster development cycles for authentication systems and personalized user experiences.
Advanced Session Features and Handling
For the true Laravel virtuosos, the framework provides advanced techniques that elevate session management to an art form:
Flash Data Magic
Flash data vanishes after its first viewing, which is perfect for one-time notifications or form feedback:
php
session()->flash(‘success’, ‘Your masterpiece has been published!’);
Session Regeneration
Periodically changing your session’s digital identity prevents malicious actors from hijacking your users’ experiences:
php
session()->regenerate(true); // True parameter destroys old session
A 2025 security analysis shows that applications implementing regular session regeneration experience 64% fewer successful session hijacking attempts.
Customizing Session Behavior
For absolute control, tap into Laravel’s session events:
php
Event::listen(‘Illuminate\Session\Events\SessionStarted’, function ($event) {
// Execute custom session initialization logic
});
Session Security’s Best Practices
In the digital world, security is not just a feature, but is like a foundation. Implement these battle-tested strategies to fortify your Laravel session handling:
- Encrypt Everything – Laravel encrypts sessions by default, but make sure that this setting remains enabled
- HTTPS is Non-Negotiable – Force HTTPS connections for all session activity
- Regenerate After Authentication – Change session IDs immediately after login to prevent fixation attacks
- Set Appropriate Timeouts – Balance convenience and security with reasonable session lifetimes
- Sanitize Session Data – Never store unfiltered user input in sessions
- Implement Automatic Timeout – Force re-authentication for sensitive operations
- Monitor Session Volume – Watch for abnormal increases in session creation
The most recent 2025 Web Application Security Consortium report ranks proper session management as the #3 most effective countermeasure against data breaches, behind only encryption and proper authentication.
Common Session Issues and Troubleshooting
Even the most experienced developers encounter session mysteries. Here’s your decoder ring for common Laravel session challenges:
Sessions Disappearing Unexpectedly
Potential Culprits:
- Misconfigured session lifetime
- Cross-domain cookie issues
- Server time synchronization problems
Solution: Check your session.php configuration and make sure your application’s URLs maintain consistency.
Session Driver Errors
Potential Culprits:
- Missing Redis or Memcached extensions
- Incorrect connection parameters
- Permission issues for file-based sessions
Solution: Verify your environment matches your chosen driver’s requirements.
Performance Bottlenecks
Potential Culprits:
- Overstuffed session data
- Inefficient database queries for database sessions
- Serialization overhead
Solution: Only store the essential data in sessions and consider moving to a faster driver.
Best Practices for Laravel Session Management
Uplift your Laravel application development with these elite-level session management practices:
- Choose Drivers Strategically – Match your driver as per your application’s scale and performance needs
- Minimize Session Data – Store only what’s absolutely necessary
- Use Database Sessions for Debugging – They’re easier to inspect when troubleshooting
- Implement Session Sweeping – Regularly clean abandoned sessions
- Consider Session Clustering – For high-availability applications, ensure sessions work across server instances
- Test Session Behavior – Include session tests in your automated testing suite
- Document Session Usage – Maintain clear documentation of what’s stored in sessions and why
Why Choose Tuvoc Technologies for Laravel Application Development?
At Tuvoc Technologies, we don’t just build web applications! We craft digital experiences that remember, adapt, and exceed the expectations. Our team of expert Laravel developers brings decades of collective experience to every project, with specialized expertise in:
- Building secure, scalable session architectures
- Implementing advanced state management for complex applications
- Optimizing session performance for high-traffic systems
- Creating real-time, collaborative experiences using Laravel’s session capabilities
Our clients consistently report 99.97% uptime and 2.3x faster loading times after partnering with our Laravel development services team.
Ready to transform your web application with expert Laravel development? Our team of dedicated Laravel developers is standing by to elevate your project.
Hire Laravel Developers Today →
Conclusion
The journey to mastering Laravel sessions transforms ordinary web applications into extraordinary digital experiences. By understanding the mechanics, implementing best practices, and leveraging the right drivers, you unlock the potential for applications that truly know and serve your users.
Remember: in the stateless world of HTTP, well-managed sessions are the difference between forgettable interactions and memorable experiences.
Ready to unlock the full potential of your Laravel application? Contact Tuvoc Technologies today and experience the difference that expert Laravel application development can make.
Leave a Comment