Understanding Functional Tests, Installing Ruby on Rails on Ubuntu, and Exploring Basic Python Codes
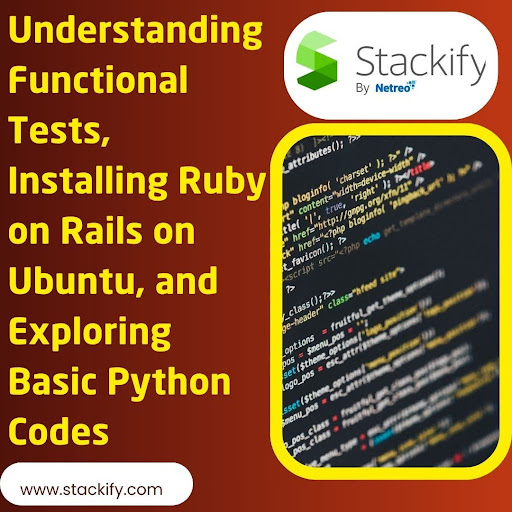
Functional testing, Ruby on Rails, and Python are foundational concepts in the world of software development. Developers often find themselves working across different languages and frameworks, making it crucial to understand these tools. In this blog, we’ll cover three important topics: functional tests, how to install Ruby on Rails on Ubuntu, and basic Python codes. By the end of this guide, you’ll have a better grasp of these essential areas, whether you’re just starting or looking to enhance your skills.
What Are Functional Tests?
Functional testing is a type of black-box testing that evaluates the functionality of a system or application. It ensures that the software behaves as expected when interacting with external systems or performing tasks. The goal of functional testing is to validate the system’s compliance with specified requirements.
Key Aspects of Functional Testing:
- User-Centric: Functional tests focus on the end-user experience by simulating real-world use cases.
- Black-Box Testing: Testers don’t need to know the internal workings of the software. They only care about inputs and outputs.
- Requirement-Based: Each test case is created based on software requirements, ensuring that all aspects of the system work as intended.
Examples of Functional Tests:
- Login Systems: Ensure that users can log in with valid credentials and are denied access with invalid credentials.
- Checkout Processes: Validate that the checkout process in an e-commerce platform follows all required steps from cart addition to payment completion.
Functional Testing vs. Unit Testing:
While unit tests verify individual components or functions of the code, functional tests assess the application as a whole. Functional testing simulates user interactions, ensuring that the application behaves correctly from a user perspective. In contrast, unit testing focuses on checking if specific functions or modules work properly.
Why Functional Testing Matters:
- Improves User Satisfaction: By catching bugs early, functional testing ensures that the user interface (UI) operates as expected.
- Ensures Compliance: Functional tests confirm that your application meets industry and security standards.
- Increases Efficiency: It reduces the risk of bugs making it into production, saving time and costs in the long run.
Ruby on Rails Ubuntu Install Guide
Ruby on Rails (RoR) is a popular framework for building web applications, especially those requiring rapid development. Installing it on Ubuntu is a straightforward process, but there are several steps to ensure that your environment is set up correctly. Let’s walk through how to install Ruby on Rails on an Ubuntu system.
Prerequisites:
- Ubuntu 20.04 or later
- Basic knowledge of the Linux terminal
Step 1: Update Your System
Before installing any packages, it’s always a good idea to update your system to ensure you have the latest versions of all packages and dependencies.
bash
Copy code
sudo apt update
sudo apt upgrade
Step 2: Install Dependencies
Ruby on Rails has several dependencies, including the database system and other development tools.
bash
Copy code
sudo apt install curl g++ git-core zlib1g-dev build-essential libssl-dev libreadline-dev libyaml-dev libsqlite3-dev sqlite3 libxml2-dev libxslt1-dev libcurl4-openssl-dev software-properties-common libffi-dev nodejs yarn
Step 3: Install rbenv and Ruby
rbenv is a tool that allows you to manage multiple Ruby versions on your system.
Install rbenv and ruby-build:
bash
Copy code
git clone https://github.com/rbenv/rbenv.git ~/.rbenv
echo ‘export PATH=”$HOME/.rbenv/bin:$PATH”‘ >> ~/.bashrc
echo ‘eval “$(rbenv init -)”‘ >> ~/.bashrc
exec $SHELL
git clone https://github.com/rbenv/ruby-build.git ~/.rbenv/plugins/ruby-build
echo ‘export PATH=”$HOME/.rbenv/plugins/ruby-build/bin:$PATH”‘ >> ~/.bashrc
exec $SHELL
Install Ruby:
bash
Copy code
rbenv install 3.0.0
rbenv global 3.0.0
Verify the Ruby installation:
bash
Copy code
ruby -v
Step 4: Install Rails
Now that Ruby is installed, the next step is to install Rails.
bash
Copy code
gem install rails -v 6.1.4
rbenv rehash
rails -v
Step 5: Install PostgreSQL (Optional)
Ruby on Rails supports multiple database systems. If you prefer using PostgreSQL, you can install it with the following command:
bash
Copy code
sudo apt install postgresql postgresql-contrib libpq-dev
Step 6: Create a New Rails Application
With Ruby on Rails installed, you can now create a new Rails application:
bash
Copy code
rails new myapp -d postgresql
Finally, navigate to your application folder and run the server:
bash
Copy code
cd myapp
rails server
Your Ruby on Rails application is now ready to be accessed at http://localhost:3000.
Basic Python Codes for Beginners
Python is a versatile and beginner-friendly programming language. Whether you’re building web applications, data analysis scripts, or automating tasks, understanding basic Python codes is essential. Here, we’ll go over some simple Python codes to get you started.
1. Hello World Program
The classic first step for any programming language is the “Hello World” program.
python
Copy code
print(“Hello, World!”)
This simple program outputs the text “Hello, World!” to the console.
2. Variables and Data Types
Python allows you to store data in variables, and it automatically assigns the data type based on the value.
python
Copy code
# Integer
x = 10
# Float
y = 3.14
# String
name = “Stackify”
# Boolean
is_active = True
3. Conditional Statements
Conditional statements are used to perform different actions based on conditions.
python
Copy code
age = 20
if age >= 18:
print(“You are an adult.”)
else:
print(“You are not an adult.”)
4. Loops
Python supports both for and while loops for repeating tasks.
For loop example:
python
Copy code
for i in range(5):
print(i)
While loop example:
python
Copy code
i = 0
while i < 5:
print(i)
i += 1
5. Functions
Functions allow you to group related code into reusable blocks.
python
Copy code
def greet(name):
return f”Hello, {name}!”
print(greet(“Stackify”))
6. Lists and Dictionaries
Python provides built-in data structures like lists and dictionaries for managing collections of data.
List example:
python
Copy code
fruits = [“apple”, “banana”, “cherry”]
print(fruits[0]) # Output: apple
Dictionary example:
python
Copy code
person = {“name”: “John”, “age”: 30}
print(person[“name”]) # Output: John
7. File Handling
Python allows you to read from and write to files.
python
Copy code
# Writing to a file
with open(‘example.txt’, ‘w’) as f:
f.write(“Hello, World!”)
# Reading from a file
with open(‘example.txt’, ‘r’) as f:
content = f.read()
print(content)
Conclusion
Functional testing, Ruby on Rails, and Python form the backbone of modern software development. Understanding the purpose and implementation of functional tests ensures that your applications meet their requirements. Installing Ruby on Rails on Ubuntu allows you to build powerful web applications quickly, while learning basic Python codes equips you with the skills needed to automate tasks and build simple programs. As you continue to develop your skills in these areas, you’ll find yourself more capable of tackling complex software challenges.
Leave a Comment